livepeer.js
Livepeer.js makes building with Livepeer effortless. It provides a core vanilla JS library to easily connect to a Livepeer provider (e.g. Livepeer Studio), as well as React-specific hooks/components to provide memoization and DOM management. Instantly build a decentralized video app with the Livepeer protocol.
npm i @livepeer/react
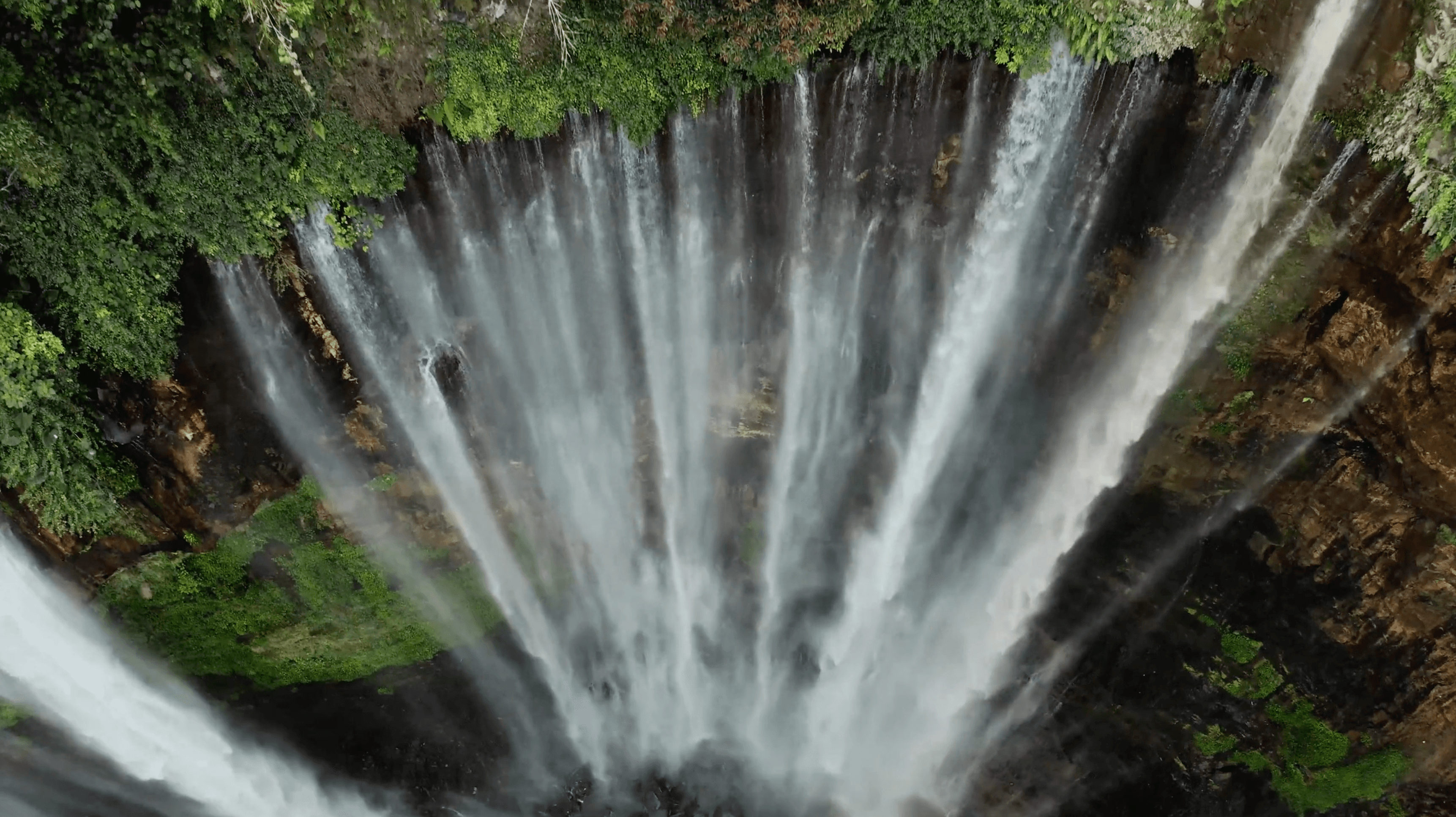
Overview
We can easily use @livepeer/react
to build a decentralized video app in minutes. First,
we create a livepeer Client
and pass it to the LivepeerConfig
React Context.
import {
LivepeerConfig,
ThemeConfig,
createReactClient,
studioProvider,
} from '@livepeer/react';
const client = createReactClient({
provider: studioProvider({ apiKey: 'yourStudioApiKey' }),
});
const livepeerTheme: ThemeConfig = {
colors: {
accent: 'rgb(0, 145, 255)',
containerBorderColor: 'rgba(0, 145, 255, 0.9)',
},
fonts: {
display: 'Inter',
},
};
function App() {
return (
<LivepeerConfig client={client} theme={livepeerTheme}>
<Livepeer />
</LivepeerConfig>
);
}
import { Player } from '@livepeer/react';
import Image from 'next/image';
import * as React from 'react';
import waterfallsPoster from '../../public/images/waterfalls-poster.png';
const PosterImage = () => {
return (
<Image
src={waterfallsPoster}
layout="fill"
objectFit="cover"
priority
placeholder="blur"
/>
);
};
const playbackId =
'bafybeigtqixg4ywcem3p6sitz55wy6xvnr565s6kuwhznpwjices3mmxoe';
export function Livepeer() {
return (
<Player
title="Waterfalls"
playbackId={playbackId}
loop
autoPlay
showTitle={false}
muted
poster={<PosterImage />}
/>
);
}
In this example, we create a livepeer Client
and pass it to the LivepeerConfig
React Context. The client is set up to use the Studio provider.
Then, we use the Player
to play a video from a playback ID (actually the IPFS CID!) with an optimized poster image! Waterfalls!
We've only scratched the surface for what you can do with livepeer.js!
Features
livepeer.js supports all these features out-of-the-box:
- Player for video/audio with built-in HLS support, accessibility, keyboard shortcuts, and customizable controls
- React hooks for working with Livepeer providers and the Livepeer protocol
- Response caching, request deduplication, and persistence
- TypeScript ready
- Tests across core and React components
...and a lot more.
Community
Check out the following places for more livepeer-related content:
- Follow @livepeer (opens in a new tab) on Twitter for project updates
- Join the discussions on GitHub (opens in a new tab)
- Jump into our Discord (opens in a new tab)